Several of our projects require back-end as well as front-end work. If a client wants us to create RESTful APIs, we start by using the OpenAPI 3 specification. We write the server code using a tool called oapi-codegen
. This article explains how we do it and add special tags to parts of our Go code, such as validate
tags.
The basic API specification
A lot of articles begin by exploring the history of the tools they discuss. Let's skip the traditional introduction and get started. At first, the API specification can be summarized as follows:
openapi: "3.0.0" info: version: 1.0.0 title: API servers: - url: http://localhost:8080/api/v1/something paths: /: post: tags: ["createSomething"] operationId: createSomething parameters: - $ref: '#/components/parameters/xForwardedFor' requestBody: content: application/json: schema: $ref: '#/components/schemas/CreateSomethingRequest' responses: '200': description: uuid of the created entity content: application/json: schema: $ref: '#/components/schemas/UUIDResponse' '401': description: unauthorized '403': description: forbidden '404': description: not found '500': description: internal server error components: schemas: CreateSomethingRequest: type: object required: - something properties: something: type: number example: 1 x-oapi-codegen-extra-tags: validate: gte=0 UUIDResponse: type: object required: - uuid properties: uuid: type: string format: uuid parameters: xForwardedFor: in: header name: X-Forwarded-For schema: type: string required: true x-oapi-codegen-extra-tags: validate
This API consists of 1 POST
request. As a request body, it has the CreateSomethingRequest
schema. We also have a header called xForwardedFor
. If you look at the definition, CreateSomethingRequest.something
and xForwardedFor
properties have a custom property called x-oapi-codegen-extra-flag
. This allows you to add extra tags to your generated struct fields. As all request bodies require validation, we chose validate
.
Now let's generate the code...
$ oapi-codegen -package main -generate types api/api.yml > openapi_types.gen.go
The generated code should be like this:
It's great to see our fields have validate
tags. Now we can begin the validation process!
The final result should look similar to what you see here, even though this is just an illustration.
Author: Csaba Ujvári
Other articles

Sep 20, 2024
Emotional Intelligence in IT Project Management
Emotional intelligence is key in IT project management. It helps leaders manage teams, resolve conflicts, and build stronger relationships, leading to more successful projects

Oct 20, 2024
Great Leaders Anticipate, Not Just React
Ready to elevate your leadership? Discover how anticipating challenges, not just reacting, can unlock success. Don't miss out on this game-changing mindset shift!...

Aug 30, 2024
How Our StemX Editors Are Revolutionizing Ticket Sales
Discover how StemX Editors are revolutionizing ticket sales with innovative design tools! See how InterTicket's new suite boosts efficiency and transforms user experiences....
AI Estimation
Let Us Provide the Perfect Solution for Your Project
Looking for accurate project estimates? Our AI-powered app helps you plan smarter. Contact us today, and we’ll provide a customized quote for your business needs — quick, reliable, and aligned with your goals.

Other articles

Sep 20, 2024
Emotional Intelligence in IT Project Management
Emotional intelligence is key in IT project management. It helps leaders manage teams, resolve conflicts, and build stronger relationships, leading to more successful projects

Oct 20, 2024
Great Leaders Anticipate, Not Just React
Ready to elevate your leadership? Discover how anticipating challenges, not just reacting, can unlock success. Don't miss out on this game-changing mindset shift!...

Aug 30, 2024
How Our StemX Editors Are Revolutionizing Ticket Sales
Discover how StemX Editors are revolutionizing ticket sales with innovative design tools! See how InterTicket's new suite boosts efficiency and transforms user experiences....
AI Estimation
Let Us Provide the Perfect Solution for Your Project
Looking for accurate project estimates? Our AI-powered app helps you plan smarter. Contact us today, and we’ll provide a customized quote for your business needs — quick, reliable, and aligned with your goals.

Other articles

Sep 20, 2024
Emotional Intelligence in IT Project Management
Emotional intelligence is key in IT project management. It helps leaders manage teams, resolve conflicts, and build stronger relationships, leading to more successful projects

Oct 20, 2024
Great Leaders Anticipate, Not Just React
Ready to elevate your leadership? Discover how anticipating challenges, not just reacting, can unlock success. Don't miss out on this game-changing mindset shift!...

Aug 30, 2024
How Our StemX Editors Are Revolutionizing Ticket Sales
Discover how StemX Editors are revolutionizing ticket sales with innovative design tools! See how InterTicket's new suite boosts efficiency and transforms user experiences....

Jun 20, 2024
Search Query Validation with React Router 6 and Yup and Typescript
Do you validate search queries in your frontend app? The answer is “sometimes”, but if you think of the search query as input for the application, you will change your mind.

Jan 28, 2024
How to Choose Between Monolithic Code and Microservices
Decipher Microservices vs. Monolithic Code: Choose wisely for a seamless development journey. Plan, embrace flexibility and avoid pitfalls. Insights from a DevOps developer.

Jan 28, 2024
Website vs Web Application: Which One Do You Need?
Decipher Microservices vs. Monolithic Code: Choose wisely for a seamless development journey. Plan, embrace flexibility and avoid pitfalls. Insights from a DevOps developer.

Sep 14, 2023
A Guide to Keeping Dependencies Up-to-Date for Enhanced Security and Efficiency in Development
Learn the importance of keeping dependencies up-to-date for a secure and efficient development process...

Sep 14, 2022
No Code Pro / Contra
No-code has caused an unusual, disruptive revelation and changes in the tech space – especially coding. Are programmers and coding still relevant or not?

Aug 14, 2022
Here are Some Reasons Why You Should Become a Software Engineer
Yes, software engineering is a good job based on virtually any criteria, including salary, the number of job openings, as well as overall job satisfaction...

Jul 14, 2022
A Guide to Working Remotely at Sea: Best Practices
Putting some effort into planning will make your entire trip more enjoyable. If you’re independently setting sail, plot a course that takes your availability into account.

Jun 14, 2022
11 Essential Skills to Become a Software Developer in 2022
Key skills for programmers and software developers to learn in 2022. If you have been doing software development for some time and thinking about what makes a good programmer?

Aug 31, 2023
The Best Way to Optimize Serverless Computing
Explore the latest features of Cloud Run for seamless deployment and scaling...

Nov 16, 2023
Discover How reCAPTCHA v3 and Cloud Armor Can Protect your Website
Discover advanced bot prevention strategies using reCAPTCHA v3 and Cloud Armor. Safeguard your website with CommIT Smart's insightful guide...

Oct 12, 2023
How to Effortlessly Automate SSL Deployment with Lego and Kubernetes
Automate SSL certificate management seamlessly with Lego and Kubernetes. Learn efficient deployment practices for a secure web environment at CommIT Smart...
AI Estimation
Let Us Provide the Perfect Solution for Your Project
Looking for accurate project estimates? Our AI-powered app helps you plan smarter. Contact us today, and we’ll provide a customized quote for your business needs — quick, reliable, and aligned with your goals.

Other articles

Sep 20, 2024
Emotional Intelligence in IT Project Management
Emotional intelligence is key in IT project management. It helps leaders manage teams, resolve conflicts, and build stronger relationships, leading to more successful projects

Oct 20, 2024
Great Leaders Anticipate, Not Just React
Ready to elevate your leadership? Discover how anticipating challenges, not just reacting, can unlock success. Don't miss out on this game-changing mindset shift!...

Aug 30, 2024
How Our StemX Editors Are Revolutionizing Ticket Sales
Discover how StemX Editors are revolutionizing ticket sales with innovative design tools! See how InterTicket's new suite boosts efficiency and transforms user experiences....
AI Estimation
Let Us Provide the Perfect Solution for Your Project
Looking for accurate project estimates? Our AI-powered app helps you plan smarter. Contact us today, and we’ll provide a customized quote for your business needs — quick, reliable, and aligned with your goals.

Other articles

Sep 20, 2024
Emotional Intelligence in IT Project Management
Emotional intelligence is key in IT project management. It helps leaders manage teams, resolve conflicts, and build stronger relationships, leading to more successful projects

Oct 20, 2024
Great Leaders Anticipate, Not Just React
Ready to elevate your leadership? Discover how anticipating challenges, not just reacting, can unlock success. Don't miss out on this game-changing mindset shift!...

Aug 30, 2024
How Our StemX Editors Are Revolutionizing Ticket Sales
Discover how StemX Editors are revolutionizing ticket sales with innovative design tools! See how InterTicket's new suite boosts efficiency and transforms user experiences....
AI Estimation
Let Us Provide the Perfect Solution for Your Project
Looking for accurate project estimates? Our AI-powered app helps you plan smarter. Contact us today, and we’ll provide a customized quote for your business needs — quick, reliable, and aligned with your goals.

Get in touch
Reach Out to Us!
Have questions or want to learn more about what we do at CommIT Smart? We’d love to hear from you! Whether you’re curious about our work or just want to start a conversation, don’t hesitate to reach out. Our team is here and ready to connect — let’s talk!
Get in touch
Reach Out to Us!
Have questions or want to learn more about what we do at CommIT Smart? We’d love to hear from you! Whether you’re curious about our work or just want to start a conversation, don’t hesitate to reach out. Our team is here and ready to connect — let’s talk!
Get in touch
Reach Out to Us!
Have questions or want to learn more about what we do at CommIT Smart? We’d love to hear from you! Whether you’re curious about our work or just want to start a conversation, don’t hesitate to reach out. Our team is here and ready to connect — let’s talk!
Get in touch
Reach Out to Us!
Have questions or want to learn more about what we do at CommIT Smart? We’d love to hear from you! Whether you’re curious about our work or just want to start a conversation, don’t hesitate to reach out. Our team is here and ready to connect — let’s talk!
Get in touch
Reach Out to Us!
Have questions or want to learn more about what we do at CommIT Smart? We’d love to hear from you! Whether you’re curious about our work or just want to start a conversation, don’t hesitate to reach out. Our team is here and ready to connect — let’s talk!
We are innovators in developing most recent web technologies, blockchain, digital technologies and apps in ingenious ways.
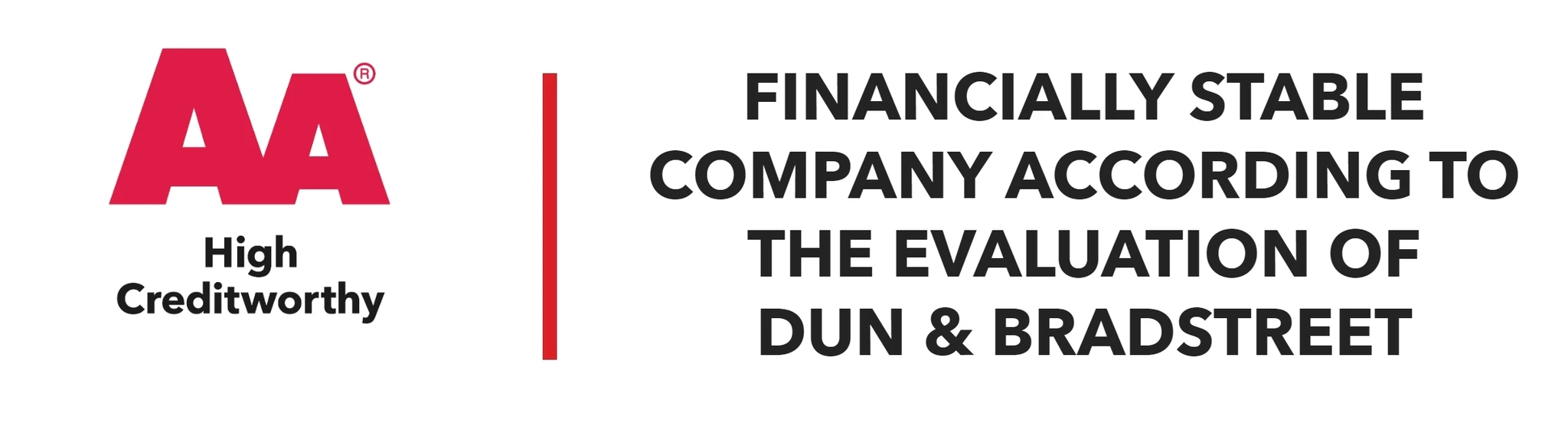
The D&B certificate is awarded exclusively to businesses with excellent creditworthiness. Possession of the certificate guarantees that establishing a business relationship with our company carries a low financial risk.
© 2024 Commitsmart Kft. All rights reserved.
We are innovators in developing most recent web technologies, blockchain, digital technologies and apps in ingenious ways.
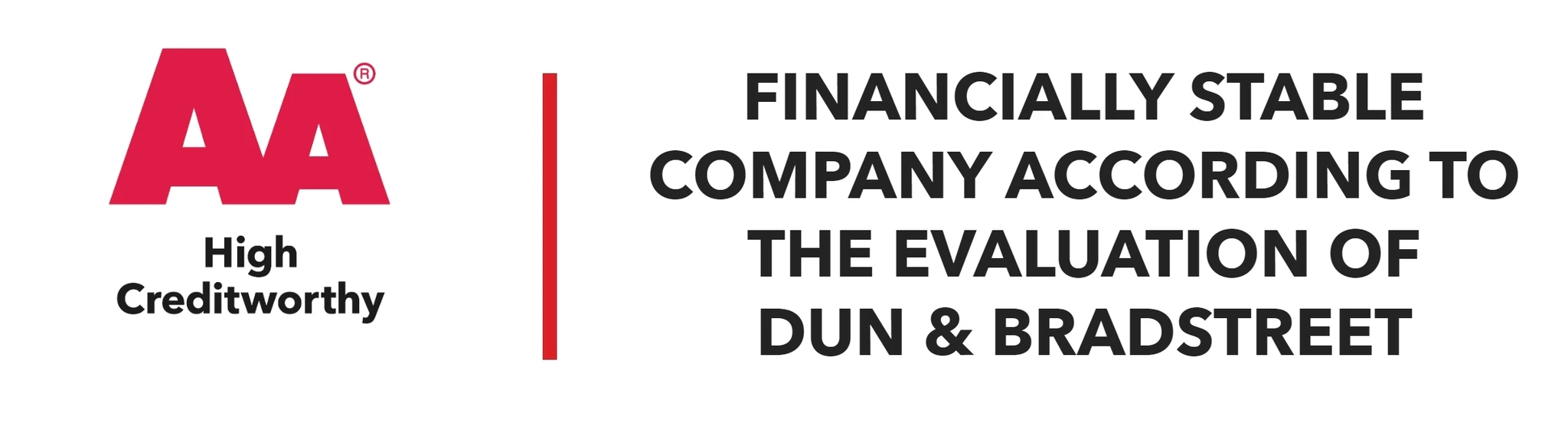
The D&B certificate is awarded exclusively to businesses with excellent creditworthiness. Possession of the certificate guarantees that establishing a business relationship with our company carries a low financial risk.
© 2024 Commitsmart Kft. All rights reserved.
We are innovators in developing most recent web technologies, blockchain, digital technologies and apps in ingenious ways.
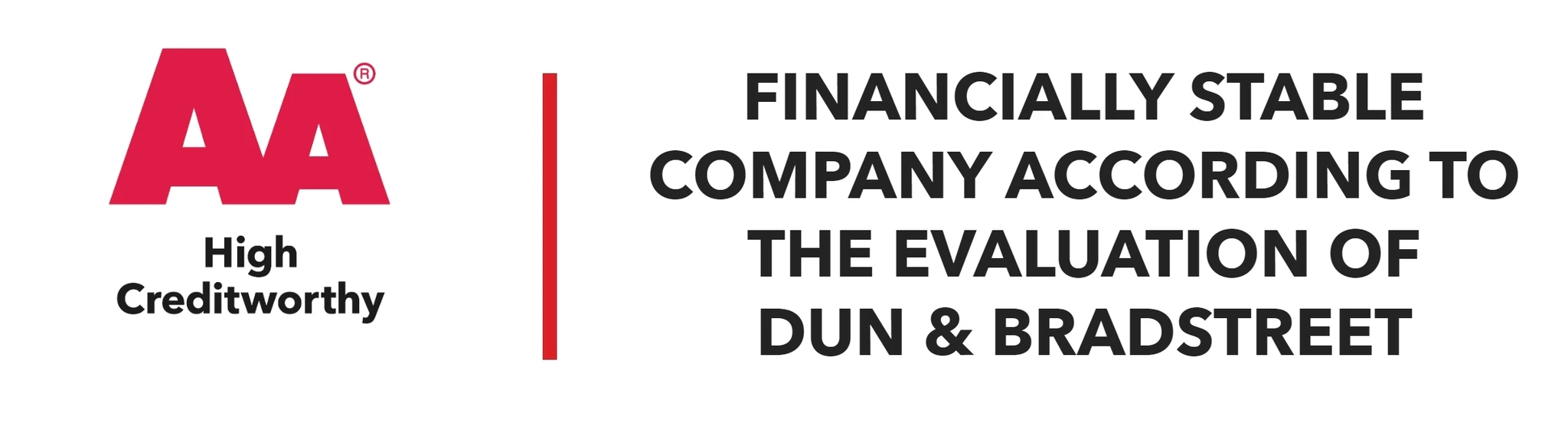
The D&B certificate is awarded exclusively to businesses with excellent creditworthiness. Possession of the certificate guarantees that establishing a business relationship with our company carries a low financial risk.
© 2024 Commitsmart Kft. All rights reserved.
We are innovators in developing most recent web technologies, blockchain, digital technologies and apps in ingenious ways.
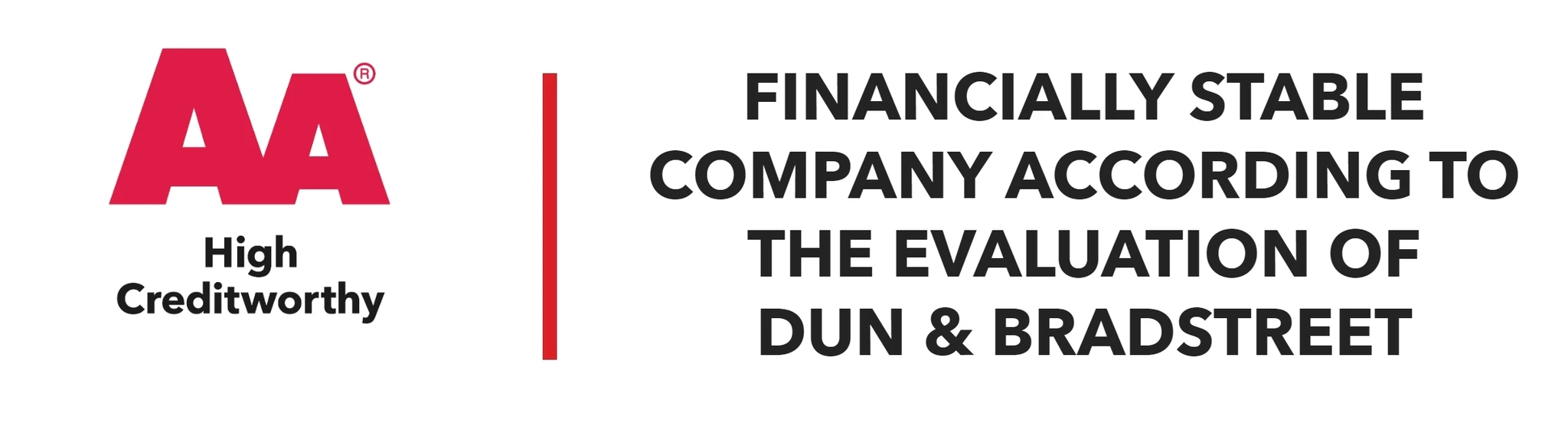
The D&B certificate is awarded exclusively to businesses with excellent creditworthiness. Possession of the certificate guarantees that establishing a business relationship with our company carries a low financial risk.
© 2024 Commitsmart Kft. All rights reserved.
We are innovators in developing most recent web technologies, blockchain, digital technologies and apps in ingenious ways.
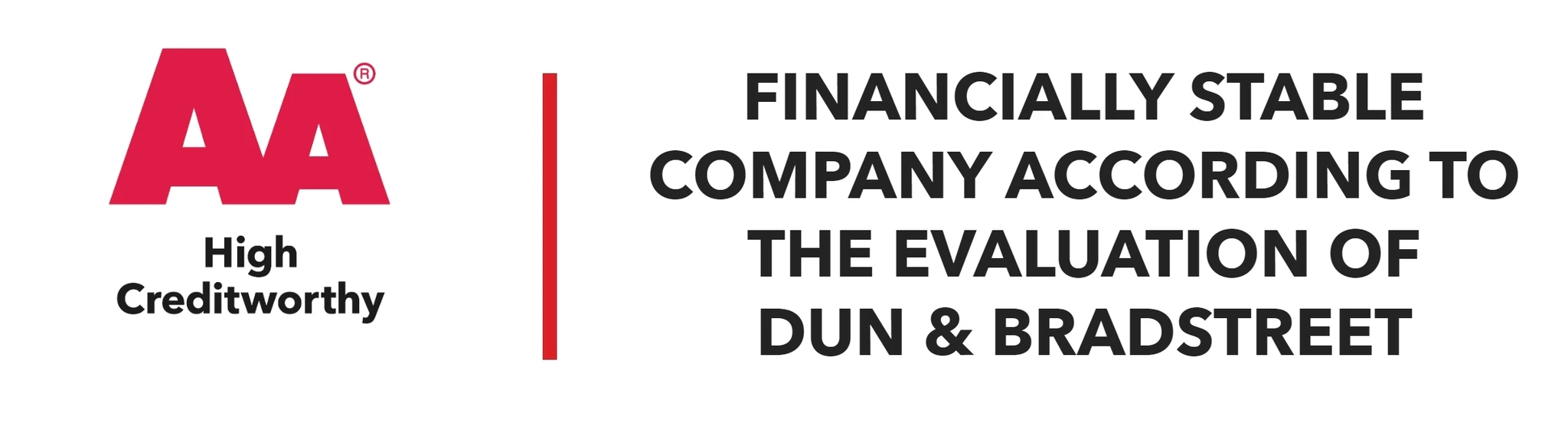
The D&B certificate is awarded exclusively to businesses with excellent creditworthiness. Possession of the certificate guarantees that establishing a business relationship with our company carries a low financial risk.
© 2024 Commitsmart Kft. All rights reserved.